- Angular is a popular open-source framework for building dynamic web applications. Angular above 2+ version is Typescript based web development framework maintained by google. with Angular we can design and create interactive single-page applications (SPAs) efficiently.
To install Angular using the Angular CLI, follow these steps:
For start Angular you need to install first Nodejs in your system
Install Node.js (if not already installed):
- We need to Download and install Node.js from nodejs.org.
- This will also install npm (Node Package Manager), which you need to install Angular.
Install the Angular CLI globally: In this step you need to install angular cli globally so Open a terminal or command prompt and run the following command:
npm install -g @angular/cli
Once the installation completes, verify it by checking the version:
ng version
Feature of Angular:
Angular have reach and powerful full feature framework for developing web-based application. Here are some of its key features:
Component-Based Architecture:
Angular is a based-on Component based architecture for building a software based on reusable part. The benefit of component based is reusable modularity stability flexibilities and maintainability.
Two-Way Data Binding:
Angular have feature of two-way data binding it means bind the data for both direction either view to controller or controller to view. two-way data binding allows automatic synchronization of data between the model (component) and the view (template). Changes in the view reflect in the model and vice versa, reducing boilerplate code for DOM manipulation.
Dependency Injection (DI):
By defining dependencies between various components and services, Agular’s DI enables developers to create code that is more modular, testable, and manageable.
Directives:
Angular provides two types of Directives that is structural (*ngIf, *ngFor) and attribute directives that allow you to manipulate the DOM. Directives let you add behaviors to elements and create custom HTML tags for reusable components.
Angular CLI:
The Angular Command Line Interface (CLI) provide a way to simplifies project setup, scaffolding, and development tasks. With commands for generating components, services, and more, it significantly accelerates the development process.
Routing:
Routing is a pattern matching technique that take incoming request from browser and map particular action or method Angular has a powerful router module for single-page applications (SPAs). It allows for defining routes, lazy loading modules, and handling navigation among different parts of the app.
Create a new Angular project
If you want to create a new Angular project, run the following command:
ng new project-name
D:\myProject\MyProjects\MyProjects\Blog\Angualr Practicle\Basic>ng new Basic
? Would you like to add Angular routing? Yes
? Which stylesheet format would you like to use? CSS
CREATE Basic/angular.json (2695 bytes)
D:\myProject\MyProjects\MyProjects\Blog\Angualr Practicle\Basic>ng new Basic
? Would you like to add Angular routing? Yes
? Which stylesheet format would you like to use? CSS
CREATE Basic/angular.json (2695 bytes)
CREATE Basic/package.json (1036 bytes)
CREATE Basic/README.md (1059 bytes)
CREATE Basic/tsconfig.json (901 bytes)
CREATE Basic/.editorconfig (274 bytes)
CREATE Basic/.gitignore (548 bytes)
CREATE Basic/tsconfig.app.json (263 bytes)
CREATE Basic/tsconfig.spec.json (273 bytes)
CREATE Basic/.vscode/extensions.json (130 bytes)
CREATE Basic/.vscode/launch.json (474 bytes)
CREATE Basic/.vscode/tasks.json (938 bytes)
CREATE Basic/src/favicon.ico (948 bytes)
CREATE Basic/src/index.html (291 bytes)
CREATE Basic/src/main.ts (214 bytes)
CREATE Basic/src/styles.css (80 bytes)
CREATE Basic/src/assets/.gitkeep (0 bytes)
CREATE Basic/src/app/app-routing.module.ts (245 bytes)
CREATE Basic/src/app/app.module.ts (393 bytes)
CREATE Basic/src/app/app.component.html (23115 bytes)
CREATE Basic/src/app/app.component.spec.ts (1070 bytes)
CREATE Basic/src/app/app.component.ts (209 bytes)
CREATE Basic/src/app/app.component.css (0 bytes)
| Installing packages (npm)...
Navigate to your project folder and start the development server:
cd project-name
ng serve
Flow of Angular Application
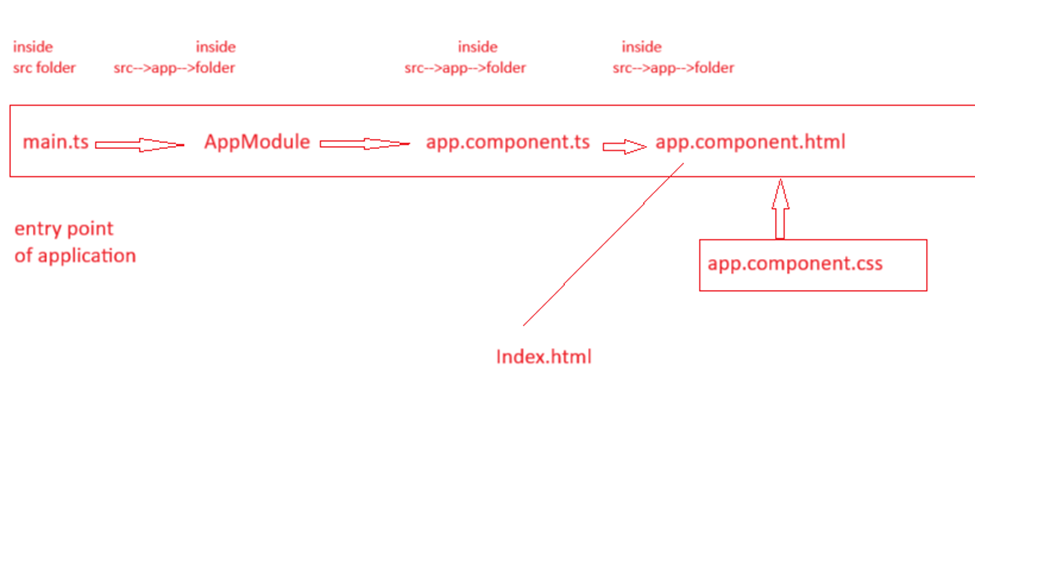
After installing Angular let discuss some main file including Entry Point of an Angular Application
That is defined under main.ts file located in the src/ directory. This file is responsible for bootstrapping the root module of the application (AppModule).
import { platformBrowserDynamic } from ‘@angular/platform-browser-dynamic’;
import { AppModule } from ‘./app/app.module’;
platformBrowserDynamic().bootstrapModule(AppModule)
.catch(err => console.error(err));
In this above code This file performs the following tasks:
- Imports the AppModule:This line of code indicate that imports the root module of the application (AppModule). AppModule is the main module that holds all the components, services, and configurations needed to run the application.
- Bootstraps the application:
This line invokes the platformBrowserDynamic() function and uses it to bootstrap (initialize) the Angular application by passing the root module AppModule.
- This is where the Angular application starts running, and the root module is loaded into the browser.
platformBrowserDynamic is responsible for compiling and bootstrapping the Angular application in a browser at runtime.
Note: This code bootstraps (starts) the Angular application using AppModule in the browser. If an error occurs during the initialization, it catches and logs the error.
Understanding AppModule (Root Module)
This module is a root module that contains declarations of components, services, and other modules that will be used in the application.
.
app.module.ts file
import { NgModule } from ‘@angular/core’;
import { BrowserModule } from ‘@angular/platform-browser’;
import { AppRoutingModule } from ‘./app-routing.module’;
import { AppComponent } from ‘./app.component’;
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
- declarations: This is section where you declare the components, directives, and pipes that belong to this module.
- imports: In This array contains other modules that are imported into this module, like BrowserModule, which is required for running the app in the browser.
- providers: Services that are provided for dependency injection.
- bootstrap: The main component that is bootstrapped when this module is loaded. In most cases, this is AppComponent
Root Component (AppComponent)
import { Component } from ‘@angular/core’;
@Component({
selector: ‘app-root’,
templateUrl: ‘./app.component.html’,
styleUrls: [‘./app.component.css’]
})
export class AppComponent {
title = ‘Basic’;
}
Key parts:
- selector: This defines the custom HTML tag (<app-root>) that represents this component in the index.html file.
- templateUrl: This links to the HTML file that defines the view for this component.
- styleUrls: This links to the CSS file(s) that define the styling for this component.
index.html
The index.html file is the main HTML file that gets served by Angular when the app is launched. It contains the root tag where the Angular app is injected.
<!doctype html>
<html lang=”en”>
<head>
<meta charset=”utf-8″>
<title>Basic</title>
<base href=”/”>
<meta name=”viewport” content=”width=device-width, initial-scale=1″>
<link rel=”icon” type=”image/x-icon” href=”favicon.ico”>
</head>
<body>
<app-root></app-root>
</body>
</html>
Bootstrapping Process
- Compilation: Angular compiles the TypeScript code and builds the application.
- Bootstrapping: Angular uses the main.ts to load the AppModule. The AppModule bootstraps the AppComponent, which is injected into the index.html via the <app-root> tag.
- Rendering: Angular dynamically updates the DOM based on the templates and component structure defined in the application.
Angular.json
Another most important file is angular.json, it is a configuration file for angular cli. Purpose of this file is it store information about the project architecture, dependencies build and test configuration and other setting. It’s generated automatically when you create a new Angular project with the Angular CLI, and it’s essential for managing the Angular workspace.
Let share Angular.json file code
{
“$schema”: “./node_modules/@angular/cli/lib/config/schema.json”,
“version”: 1,
“newProjectRoot”: “projects”,
“projects”: {
“Basic”: {
“projectType”: “application”,
“schematics”: {},
“root”: “”,
“sourceRoot”: “src”,
“prefix”: “app”,
“architect”: {
“build”: {
“builder”: “@angular-devkit/build-angular:browser”,
“options”: {
“outputPath”: “dist/basic”,
“index”: “src/index.html”,
“main”: “src/main.ts”,
“polyfills”: [
“zone.js”
],
“tsConfig”: “tsconfig.app.json”,
“assets”: [
“src/favicon.ico”,
“src/assets”
],
“styles”: [
“src/styles.css”
],
“scripts”: []
},
“configurations”: {
“production”: {
“budgets”: [
{
“type”: “initial”,
“maximumWarning”: “500kb”,
“maximumError”: “1mb”
},
{
“type”: “anyComponentStyle”,
“maximumWarning”: “2kb”,
“maximumError”: “4kb”
}
],
“outputHashing”: “all”
},
“development”: {
“buildOptimizer”: false,
“optimization”: false,
“vendorChunk”: true,
“extractLicenses”: false,
“sourceMap”: true,
“namedChunks”: true
}
},
“defaultConfiguration”: “production”
},
“serve”: {
“builder”: “@angular-devkit/build-angular:dev-server”,
“configurations”: {
“production”: {
“browserTarget”: “Basic:build:production”
},
“development”: {
“browserTarget”: “Basic:build:development”
}
},
“defaultConfiguration”: “development”
},
“extract-i18n”: {
“builder”: “@angular-devkit/build-angular:extract-i18n”,
“options”: {
“browserTarget”: “Basic:build”
}
},
“test”: {
“builder”: “@angular-devkit/build-angular:karma”,
“options”: {
“polyfills”: [
“zone.js”,
“zone.js/testing”
],
“tsConfig”: “tsconfig.spec.json”,
“assets”: [
“src/favicon.ico”,
“src/assets”
],
“styles”: [
“src/styles.css”
],
“scripts”: []
}
}
}
}
},
“cli”: {
“analytics”: “a91b3513-1f69-41e2-92da-53dd9f0a5ffc”
}
}
- projects
- The In this projects section lists all applications and libraries in the Angular application workspace.
- where Each project contain its own set of configuration sections, typically including the main application (usually named the same as the project) and any additional apps or libraries.
“projects”: {
“my-app”: { … },
“my-library”: { … }
}
- architect
In this section I
nside each project, the architect property defines build, serve, test, and lint options for the project. This includes settings for:
- Build: Defines how the application should be built, including options for AOT, optimization, output paths, etc.
- Serve: Settings for running a local development server.
- Test: Configuration for running tests.
- Lint: Configuration for code linting.
“architect”: {
“build”: { … },
“serve”: { … },
“test”: { … },
“lint”: { … }
}
3 buildOptions
Located inside the build configuration under architect, it specifies options for building the app.
- Key settings include:
- outputPath:This outPath Specifies where compiled files are stored (e.g., dist/my-app).
- index, main, polyfills: Paths to main files (e.g., src/index.html, src/main.ts, etc.).
- assets, styles, scripts: Lists of asset files, global stylesheets, and scripts to include
“options”: {
“outputPath”: “dist/basic”,
“index”: “src/index.html”,
“main”: “src/main.ts”,
“polyfills”: [
“zone.js”
],
“tsConfig”: “tsconfig.app.json”,
“assets”: [
“src/favicon.ico”,
“src/assets”
],
“styles”: [
“src/styles.css”
],
“scripts”: []
},
- configurations In the angular.json file, the configurations section under build allows you to define environment-specific build configurations
“configurations”: {
“production”: {
“budgets”: [
{
“type”: “initial”,
“maximumWarning”: “500kb”,
“maximumError”: “1mb”
},
{
“type”: “anyComponentStyle”,
“maximumWarning”: “2kb”,
“maximumError”: “4kb”
}
],
“outputHashing”: “all”
},
“development”: {
“buildOptimizer”: false,
“optimization”: false,
“vendorChunk”: true,
“extractLicenses”: false,
“sourceMap”: true,
“namedChunks”: true
}
},
- budgets: Sets file size limits for the build to help keep bundle sizes optimized. If these limits are exceeded, Angular will issue warnings or errors.
- initial: Sets limits on the initial bundle size (in this case, 500KB for warnings, 1MB for errors).
- anyComponentStyle: Sets limits for individual component stylesheets (in this case, 2KB for warnings, 4KB for errors).
- outputHashing: Adding hash values to filenames (“all” applies hashing to all output files) for cache-busting. This is important for production as it ensures users always get the latest version when files change.
- buildOptimizer: Improves build size and performance by removing unnecessary code. In development, it’s often set to false to speed up build times.
- optimization: This controls whether production-level optimizations (e.g., minification, tree shaking) are enabled. false in development helps keep the code readable and debuggable.
- vendorChunk: When set to true, Angular separates vendor libraries (like Angular itself, RxJS) into a separate chunk, which can speed up rebuilds during development.
- extractLicenses: If true, Angular extracts licenses from third-party libraries into a separate file. Often turned off in development to speed up build times.
- sourceMap: Enables source maps, allowing you to view original TypeScript code in the browser’s developer tools for easier debugging.
- namedChunks: If true, Angular gives chunks readable names, making it easier to identify them in the build output, useful for debugging during development
Package.Json File
Package .json hold all of the NPM package installed for Project.
Package.json file locates in project root and contains information about your web application. The main purpose of this file is containing the information about npm packages installed for the project.
{
“name”: “basic”,
“version”: “0.0.0”,
“scripts”: {
“ng”: “ng”,
“start”: “ng serve”,
“build”: “ng build”,
“watch”: “ng build –watch –configuration development”,
“test”: “ng test”
},
“private”: true,
“dependencies”: {
“@angular/animations”: “^15.1.0”,
“@angular/common”: “^15.1.0”,
“@angular/compiler”: “^15.1.0”,
“@angular/core”: “^15.1.0”,
“@angular/forms”: “^15.1.0”,
“@angular/platform-browser”: “^15.1.0”,
“@angular/platform-browser-dynamic”: “^15.1.0”,
“@angular/router”: “^15.1.0”,
“rxjs”: “~7.8.0”,
“tslib”: “^2.3.0”,
“zone.js”: “~0.12.0”
},
“devDependencies”: {
“@angular-devkit/build-angular”: “^15.1.4”,
“@angular/cli”: “~15.1.4”,
“@angular/compiler-cli”: “^15.1.0”,
“@types/jasmine”: “~4.3.0”,
“jasmine-core”: “~4.5.0”,
“karma”: “~6.4.0”,
“karma-chrome-launcher”: “~3.1.0”,
“karma-coverage”: “~2.2.0”,
“karma-jasmine”: “~5.1.0”,
“karma-jasmine-html-reporter”: “~2.0.0”,
“typescript”: “~4.9.4”
}
}
{
“name”: “basic “,
“version”: “0.0.0”,
“description”: “An Angular project”,
“author”: “Apitpoint”,
“license”: “MIT”
}
- name: The project’s name (should be unique across npm).
- version: The current version, often following semantic versioning.
- description: A short description of the project.
- author: The creator of the project.
- license: Specifies the type of license (e.g., MIT, ISC).
Script
This section describes about Node scripts you can run your application
“scripts”: {
“ng”: “ng”,
“start”: “ng serve”,
“build”: “ng build”,
“watch”: “ng build –watch –configuration development”,
“test”: “ng test”
},
- start: Runs ng serve to start a development server.
- build: Runs ng build to build the project.
- test: Runs tests.
3. Dependencies
- Lists the packages that the project needs to run. Each dependency specifies a version range for npm to install.
“dependencies”: {
“@angular/animations”: “^15.1.0”,
“@angular/common”: “^15.1.0”,
“@angular/compiler”: “^15.1.0”,
“@angular/core”: “^15.1.0”,
“@angular/forms”: “^15.1.0”,
“@angular/platform-browser”: “^15.1.0”,
“@angular/platform-browser-dynamic”: “^15.1.0”,
“@angular/router”: “^15.1.0”,
“rxjs”: “~7.8.0”,
“tslib”: “^2.3.0”,
“zone.js”: “~0.12.0”
},
Lists the packages that the project needs to run. Each dependency specifies a version range for npm to install.
4. DevDependencies
- These packages are only needed during development, not for production:
“devDependencies”: {
“@angular-devkit/build-angular”: “^15.1.4”,
“@angular/cli”: “~15.1.4”,
“@angular/compiler-cli”: “^15.1.0”,
“@types/jasmine”: “~4.3.0”,
“jasmine-core”: “~4.5.0”,
“karma”: “~6.4.0”,
“karma-chrome-launcher”: “~3.1.0”,
“karma-coverage”: “~2.2.0”,
“karma-jasmine”: “~5.1.0”,
“karma-jasmine-html-reporter”: “~2.0.0”,
“typescript”: “~4.9.4”
}