Introduction to ASP.NET Core
Now a days every one talk about open source cross platform application development. Microsoft .net framework is always knows for windows-based application, it means once you create a application we can run only windows based operation system. .NET Core is the new open-source and cross-platform framework to build applications for all operating systems including Windows, Mac, and Linux.
• There is Built-in supports for Dependency Injection.
• There is no web.config file. We can store the custom configuration into an appsettings.json file.
• There is no Global.asax file. We can now register and use the services in the startup class.
• It has good support for asynchronous programming
• .NET core Supports Multiple Platforms
• IoC Container
• Integration with Modern UI Frameworks like Angular React etc.
For more details, you can follow these blog links :
“Startup“
Bellow .NET core 6 there is startup class. It is the entry point of the ASP.NET Core mvc application. Every .NET Core application must have this class. This class contains the application configuration related items. It is not necessary that the class name must be “Startup”, it can be anything, we can configure the startup class in the Program class.
ConfigureServices() : The ConfigureServices function is a place allows you to register your dependent classes with the built-in IoC container. After registering the dependent class, it can be utilized throughout the application.
Configure():The Configure method is a place where we can configure the application request pipeline for our asp.net core application using the IApplicationBuilder instance that is provided by the built-in IoC container. ASP.NET Core introduced the middleware components to define a request pipeline, which will be executed on
every request.
IApplicationBuilder is an interface that includes attributes and methods specific to the present environment. It retrieves the application’s environment variables.
IHostingEnvironment is an interface that contains information related to the web hosting environment on which application is running. Using this interface method, we can change behavior of application.
What is MVC
MVC stand for Model View Controller it is architectural design pattern primarily Used in web application.
1 Model:
Role: the primary role of model is handle business logic that represent the data and business logic of the application
Responsibilities:
- Handel the data storage retrieval and manipulation of mainly interacting with a database
- Contains the core logic for managing application rules, validations, and operations.
Example: Suppose there is a application like an eCommerce app, a Product model could represent the data related to products, like ID, Name, Price, and Stock, along with business logic such as applying discounts or checking availability.
2. View:
Role:
primary role of this views is representing data. he View is responsible for the presentation layer or UI of the application. It displays data to the user and renders the interface elements like forms, lists, and buttons.
Responsibilities:
- Receives data from the Model (via the Controller) and displays it to the user.
- Ensures that the UI is updated according to the data changes in the Model.
Example: In the eCommerce app, the View could be an HTML page or a component that shows a list of products, with prices and descriptions, allowing users to browse and select items3:
Controller
Role: controller is handled user request with the system acting as a mediator between the View and Model.
Responsibilities:
- Processes input (such as clicks or form submissions) from the user and determines how the application should respond.
- Manipulates the Model based on user actions or commands.
Example: When a user clicks “Add to Cart” in an eCommerce app, the Controller will process this action, call the Model to update the cart, and then refresh the View to show the updated cart contents
Key Benefits of MVC:
Key Benefits of MVC:
Separation of concerns: separation of concern is a process that divides a program into sections, each addressing a separate concern. Then in term of MVC Each component (Model, View, Controller) has a clear responsibility, making the code more modular and maintainable.
Testability:
It is simpler to create unit tests for distinct components when the logic and UI are kept apart.
Scalability: The separation allows easier modification and extension of the codebase as the application grows.
Understanding the Project Structure
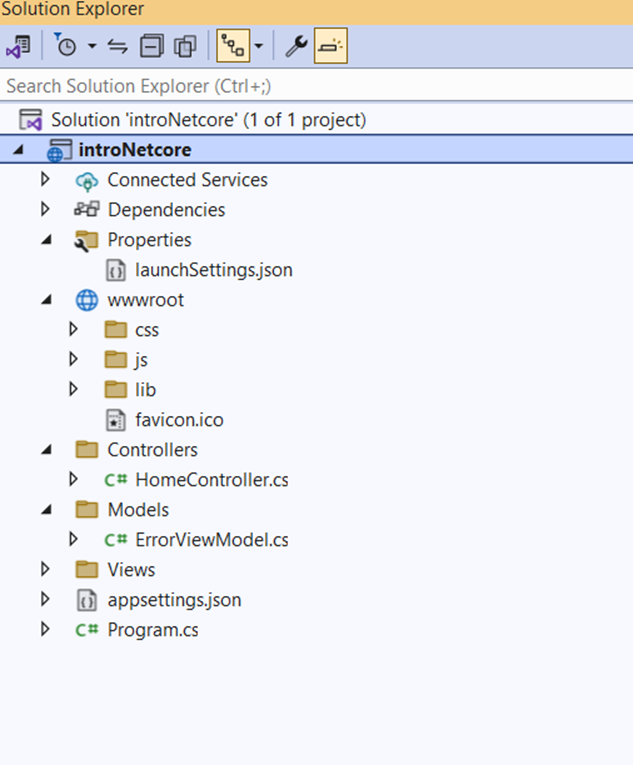
1. Solution File (.sln)
- The .sln file represents the entire solution in Visual Studio, containing information about the included projects and their configurations.
2. Project Root Folder
Contains key folders and configuration files for the ASP.NET Core MVC project.
3. Controllers Folder
- Purpose: Controlle take a input request and respond particular Action Method This classes, responsible for handling HTTP requests and returning responses.
- Example: HomeController.cs, SecurityController.cs
4. Models Folder
- Purpose: It Stores model classes, representing the data and business logic.
- Example: Product.cs, Customer.cs
5. Views Folder
- Purpose: Stores view files (UI) in .cshtml format that render HTML/CSS to display data to the user.
- Example: Views/Home/Index.cshtml, Views/Shared/_Layout.cshtml
6. wwwroot Folder
- Purpose: www folder contain static file it contain important static file such as css js etc
- Example: wwwroot/css/site.css, wwwroot/js/site.js
7. Program.cs and Startup.cs
- Program.cs: Program.cs file is entry point of the application. Defines the hosting and configuration for the app.
- Startup.cs: Configures services and middleware, handling the application’s HTTP request pipeline.
8. AppSettings.json
- Purpose: The appsettings.json file is an application configuration file used to store configuration settings such as
database connections strings,any application scope global variables, etc - Example: appsettings.json for storing connection strings, logging settings, etc.
9. Properties Folder (focus on launchSettings.json)
- Purpose: The launchSettings.json file is used to store the configuration information, which describes how to start the
ASP.NET Core
application, using Visual Studio. The file is used only during the development of the application using VisualStudio.
It contains only those settings that required to run the application.
The file has two sections. One is iisSettings and the other one is profiles section
iisSettings: contains the settings required to debug the application under the IIS or IIS Express
….profiles section contains the debug profiles
Routing in ASP.NET Core MVC
Routing is the pattern matching system. Routing map the incoming HTTP request to appropriate controller actions.
Types of Routing in ASP.NET Core MVC
There are two main types of routing in ASP.NET Core MVC:
- Default Routing (Convention-Based Routing)
- Attribute Routing
Convention-Based Routing
In convention-based routing eliminates the need to explicitly define routes on each action or controller by mapping URL patterns to controller actions.in .net core MVC when user request any page ,the request URL should match with particular URL pattern and it decides what to do with that incoming Http request. This is defined in the Startup.cs file within the Use Endpoints or UseMvc in Configure method in below .NET core 6
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: “default”,
pattern: “{controller=Home}/{action=Index}/{id?}”);
});
Pattern Explanation:
{controller=Home}: The controller part of the URL. Defaults to the HomeController if not provided.
{action=Index}: The action method within the controller. Defaults to the Index action if not provided.
{id?}: An optional parameter, typically used to identify resources (e.g., id=1).
in above .NET core 6
app.MapControllerRoute(
name: “default”,
pattern: “{controller=Home}/{action=Index}/{id?}”);
Attribute based Routing:
Attribute based routing is a technique allows to developer to define route directly on controller action or at the controller level using attribute symbolled [].
This gives you more control over the routing and is useful for complex scenarios where convention-based routing is not sufficient.
[Route(“product”)]
public class ProductController : Controller
{
[Route(“details/{id}”)]
public IActionResult Details(int id)
{
return View();
}
}
/product/details/5 → Matches the Details action in ProductsController and passes 5 as the id.
Route Parameters and Constraints
Route Parameters:
Route parameters allow dynamic values to be passed in the URL, making the routing system more flexible.
Example of Route Parameters:
[Route(“productPage/{id}”)]
public IActionResult Details(int id)
{
return View();
}
The {id} is a route parameter that will capture part of the URL and pass it to the action method.
Example URL:
/productPage/1 → Calls the Details action with id = 1.
Route Constraints:
Route constraints allow you to restrict the types or formats of values that can be passed to a route parameter.
Example of Route Constraints:
[Route(“products/{id:int}”)]
public IActionResult Details(int id)
{
// Logic to handle the product based on the id parameter
return View();
}
The {id:int} constraint ensures that the id parameter must be an integer
Advanced Features of Routing
Route Data Tokens: Provide additional metadata or custom data along with routes.
Route Priority: In case of multiple matching routes, priority can be controlled.
Catch-All Parameters: Can be used to capture the remainder of a URL (e.g., {*slug}).
Example of Catch-All:
[Route(“blog/{*slug}”)]
public IActionResult Blog(string slug)
{
// Handle the blog post with the given slug
return View();
}
The URL /blog/my-first-post would pass my-first-post as the slug parameter.
Partial Views in ASP.NET Core MVC
A Partial View in ASP.NET Core MVC is special type of views which is render a portion of views contains. It help us to reduce code duplication. It allows developers to break down a large view into smaller, manageable parts that can be reused across multiple views. Partial views help keep code organized, reduce duplication, and make it easier to maintain the application.
When to Use Partial Views:
- Reuse Common UI Elements: When you have shared elements such as headers, footers, navigation menus, etc., that appear on multiple pages.
- Modularize Views: To break down complex views into smaller, maintainable parts.
- Update a Section of a Page: Partial views can be used in combination with AJAX to update specific sections of a page dynamically without refreshing the whole page.
In ASP .NET ore MVC there are many ways to render and use partial views. You can either use the <partial>
tag helper, Html.PartialAsync
or Html.RenderPartialAsync
methods, or even ViewComponent
. Here are the ways to use partial views:
- Using
<partial>
Tag Helper
This is easiest and most modern way to render a partial view in Razor is by using the <partial>
tag helper
<partial name=”_UsersPartial” model=”Model.Users” />
name: Specifies the name of the partial view (in this case _UsersPartial).
model: Optional, you can pass the model that the partial view expects.
- Using
Html.PartialAsync
(Asynchronous)
@await Html.PartialAsync(“_UsersPartial”, Model.Users)
3. Using Html.RenderPartialAsync
(Asynchronous with Direct Output)
Html.RenderPartialAsync
directly writes the content to the output stream instead of returning an IHtmlContent
, making it slightly faster but less flexible
@{ await Html.RenderPartialAsync(“_UsersPartial”, Model.Users); }
Example: How we can use partial view
Supposes We want to display a list of products on a page. Each product will be rendered using a partial view to keep the view clean and reusable.
Create a Model
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
Step 2: Create the Partial View
Create a partial views to display a product details
In the Views/Shared
folder (or any other appropriate folder), create a partial view named _ProductPartial.cshtml
You can create a partial view any of views folder but in this example we are creating under shared folder.
Named ProductPartial
<!– Views/Shared/_ProductPartial.cshtml –>
@model MyApp.Models.Product
<div class=”product-card”>
<h1>This is example of Product details partial views </h1>
<h3>@Model.Name</h3>
<p>Price: $@Model.Price</p>
</div>
Step 3: Create the Controller
Next we need to create a controller to handel views .
// Controllers/ProductController.cs
using Microsoft.AspNetCore.Mvc;
using MyApp.Models;
public class ProductController : Controller
{
public IActionResult Index()
{
// Sample list of products
var products = new List<Product>
{
new Product { Id = 1, Name = “TV”, Price = 999.99m },
new Product { Id = 2, Name = “Smartphone”, Price = 499.99m },
new Product { Id = 3, Name = “Laptop”, Price = 299.99m }
};
return View(products);
}
}
Step 4: Create the Parent View
In the Views/Product
folder, create a view named Index.cshtml
.
<!– Views/Product/Index.cshtml –>
@model List<MyApp.Models.Product>
<h1>Product List</h1>
@foreach (var product in Model)
{
<!-- Render the partial view for each product -->
<partial name="_ProductPartial" model="product" />
}
Tag Helpers in ASP NET CORE
When you create a webpage or form using HTML then it sis client side this approach is client side But when you use Tag helper then this approach is server side . it is basically special type attribute provide by ASP .net core. Tag Helpers are components that enable server-side code to participate in creating and rendering HTML elements in Razor views.
Note: before start tag helper make sure you have include name space for tag helpers in your viewImports file.
Microsoft.AspNetCore.Mvc.TagHelps.
Add this line in your ViewImports file.
@addTagHelper*,
Microsoft.AspNetCore.Mvc.TagHelprs
Why Use Tag Helpers?
Tag Helpers provide several benefits:
- Readable HTML-like syntax: They make Razor markup more readable by resembling standard HTML, enhancing the development experience.
- Intellisense support: IDEs like Visual Studio provide better tooling and Intellisense support for Tag Helpers, making development faster and less error-prone.
- Simplifies complex logic: Common tasks like form generation, linking, and validation are easier with Tag Helpers
Form-Related Tag Helpers
<form>
Tag Helper
- Used to bind a form to an action in a controller.
- Attributes:
asp-action
: This is Specifies the controller action.asp-controller
: This is Specifies the controller.asp-route-{parameter}
: Specifies route parameters.
<form asp-controller=”Home” asp-action=”Submit”>
2 <input> Tag Helper
- Used to create input elements in a form.
- Attributes:
- asp-for:This is used to Binds to a model property.
- type: This is Specifies input type (text, email, password, etc.)
<input asp-for=”Email” type=”email” />
3 <textarea> Tag Helper
- Generates a textarea for multiline text input.
- Attributes:
- asp-for: Binds to a model property.
<textarea asp-for=”Comments”></textarea>
4 <select> Tag Helper
- Used to create a drop-down list (select box).
- Attributes:
- asp-for: Binds to a model property.
- asp-items: Specifies a list of items to populate the dropdown.
<select asp-for=”Country” asp-items=”Model.Countries”></select>
5 <label> Tag Helper
- Generates a label for a form field.
- Attributes:
Asp-for
<label asp-for=”Name”></label>
6 <button> Tag Helper
- Generates a button element.
- Attributes:
- asp-action: This Specifies the action method.
- asp-controller: This Specifies the controller.
Example:
<button type=”submit” asp-action=”Save”>Save</button>
Link and URL-Related Tag Helpers
- Anchor Tag Helper (
<a>
)
- Generates a hyperlink based on routing.
- Attributes:
asp-action
: Specifies the action method.asp-controller
: Specifies the controller.asp-route-{parameter}
: Specifies route parameters.
Example:
html
Copy code
<a asp-controller="Product" asp-action="Details" asp-route-id="5">View Details
</a>
- Link Tag Helper (
<link>
)
- IT is Used for linking to external CSS files.
- Attributes:
href
: URL to the resource (automatically resolved).
Example:
html
<link rel="stylesheet" href="~/css/site.css" />
- Script Tag Helper (
<script>
)
- This Used for linking to JavaScript files.
- Attributes:
src
: URL to the resource (automatically resolved).
Example:
html
Copy code
<script src="~/js/site.js"></script>
Validation Tag Helpers
- Validation Message Tag Helper (
<span>
)
- Displays validation messages for a specific field.
- Attributes:
asp-validation-for
: Binds to a model property.
<span asp-validation-for=”Email” class=”text-danger”></span>
Validation Summary Tag Helper (<div>
)
Displays a summary of validation errors.
Attributes:
- asp-validation-summary: Set to All or ModelOnly.
<div asp-validation-summary=”All” class=”text-danger”></div>
Environment Tag Helper
- Environment Tag Helper (
<environment>
)
- This is used for Renders different content based on the environment (Development, Staging, Production).
- Attributes:
include
: Environment(s) where content should be rendered.exclude
: Environment(s) where content should not be rendered.
Example:
<environment include=”Development”>
<link rel=”stylesheet” href=”~/lib/bootstrap/bootstrap.css” />
</environment>
<environment exclude=”Development”>
<link rel=”stylesheet” href=”~/lib/bootstrap/bootstrap.min.css” />
</environment>
@model YourApp.Models.UserRegistrationModel
@{
ViewData["Title"] = "User Registration";
}
<h2>User Registration</h2>
<!-- Display validation summary -->
@if (ViewData.ModelState.IsValid == false)
{
<div class="text-danger">
@Html.ValidationSummary()
</div>
}
<form asp-action="Register" method="post">
<div class="form-group">
<label asp-for="FirstName"></label>
<input asp-for="FirstName" class="form-control" />
<span asp-validation-for="FirstName" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="LastName"></label>
<input asp-for="LastName" class="form-control" />
<span asp-validation-for="LastName" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Email"></label>
<input asp-for="Email" class="form-control" />
<span asp-validation-for="Email" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Password"></label>
<input asp-for="Password" type="password" class="form-control" />
<span asp-validation-for="Password" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="ConfirmPassword"></label>
<input asp-for="ConfirmPassword" type="password" class="form-control" />
<span asp-validation-for="ConfirmPassword" class="text-danger"></span>
</div>
<button type="submit" class="btn btn-primary">Register</button>
</form>
@section Scripts {
<!-- Include client-side validation scripts -->
<partial name="_ValidationScriptsPartial" />
Action Method and Action Result in MVC
Action method means respond user request, controller method that respond to various HTTP Verbs like Get ,Post,Put,Delete.
- Action method must be public
- It can not be static.
- It must return a value.
- It must be defined a return type
- It can not be void.
- It can be parametrized or parameter less.
- It can not have ref and out parameter.
- It can overload.
- It cannot be override.
Action Result:
Action result in MVC defined framework level operation. It encapsulate the result of an action method . ActionResult in MVC plays a key role in abstracting different types of responses, including rendering views, redirecting requests, returning data in various formats (like JSON or XML), or simply sending HTTP status codes.
Types of Action Results:
ViewResult
PartialViewResult
FileResult
JSON Result
Content Result
Redirect Result RedirectTo routresult
Middleware in ASP.NET CORE
Middleware is a piece of code in an application pipeline used to handle requests and responses.
Middleware is fundamental to the ASP.NET Core architecture, and it plays a key role in processing every request that enters the application and in generating the response.
Each middleware component in the pipeline can:
- Handle the request: Perform some operation and pass control to the next component in the pipeline.
- Generate a response: Return a response directly, short-circuiting the remaining middleware.
For example, we may have a middleware component to authenticate a user, another piece of middleware to handle errors, and another middleware to serve static files such as JavaScript files, CSS files, images, etc.
Built-in Middleware Examples:
ASP.NET Core provides several built-in middleware components, such as:
- Routing: Defines request routing to match specific endpoints.
- Static Files: Serves static files like CSS, JavaScript, and images.
- Authentication: Handles user authentication.
- Session: Manages user session state.
- CORS: Allows cross-origin requests.
- Exception Handling: Handles global exceptions and errors.
General Order of Middleware:
· Exception Handling Middleware
- Capture and handle exceptions that occur in subsequent middleware.
- Example: app.UseExceptionHandler(“/Home/Error”);
· Static Files Middleware
- Serve static files like images, CSS, and JavaScript from the wwwroot folder.
- Example: app.UseStaticFiles();
· Routing Middleware
- Enable routing capabilities to match incoming requests to endpoints.
- Example: app.UseRouting();
· Authentication Middleware
- Authenticate users and populate the HttpContext.User with claims.
- Example: app.UseAuthentication();
· Authorization Middleware
- Authorize users based on claims or policies defined in your application.
- Example: app.UseAuthorization();
· Session Middleware (if using)
- Manage user session state, allowing for data to persist across requests.
- Example: app.UseSession();
· Custom Middleware (if any)
- Any user-defined middleware for specific functionalities, such as logging or performance monitoring.
- Example: app.UseMiddleware<CustomMiddleware>();
· Endpoints Middleware
- Map incoming requests to controller actions or Razor pages. This is where the endpoint routing happens.
- Example: app.UseEndpoints(endpoints => { endpoints.MapControllers(); });
Run(): This method is used to complete the middleware execution
Next() This method is used to pass the execution to next middleware.
Use(); this method is used to insert new middleware.
Map(): this method is used to map the middleware to specific url.
For more details please visit:
APITPOINT Websitefor featured image : freepik.com